Build 1 - Player Movement and Example Scene
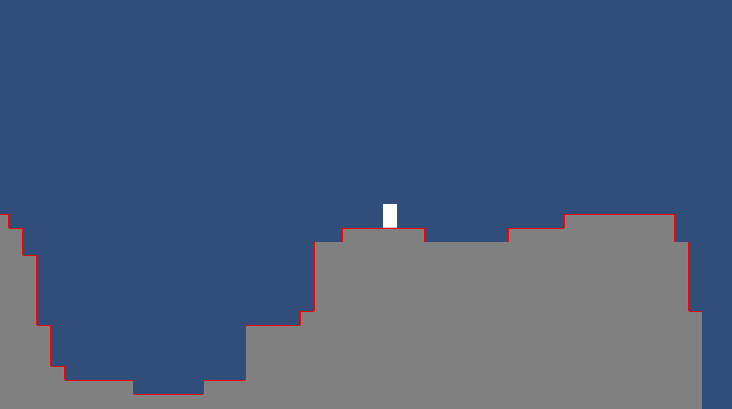
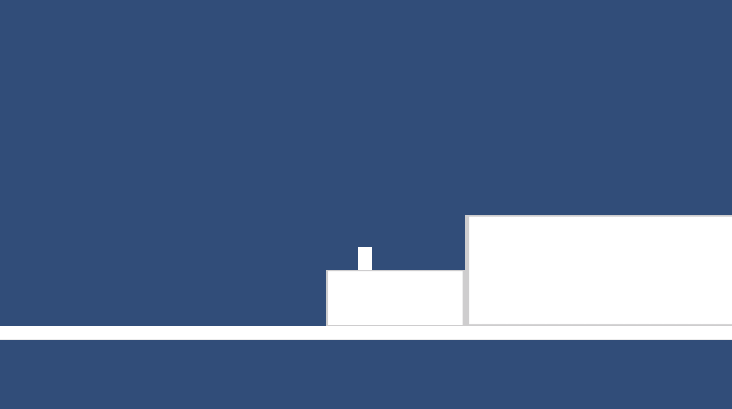
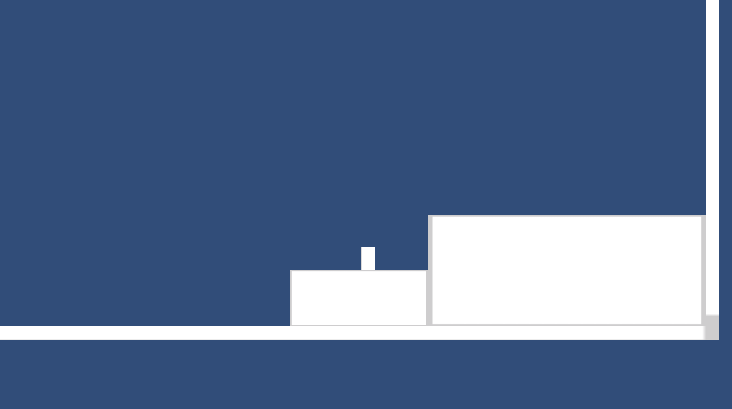
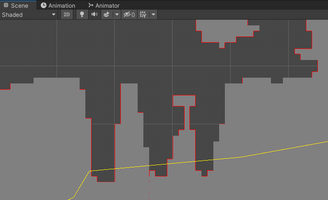
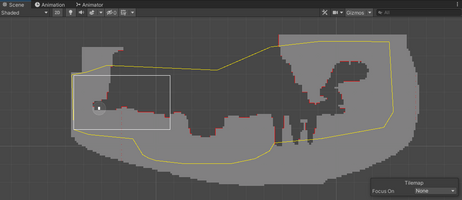
The First Build
The first build of this game shows off the basic movement for the player character in the game, as well as a scene that in the future would act as a tutorial level for the player to get used to the movement of the character.
Player Movement
In the current build, the player movement is fairly simple. A and D move the player left and right respectively, and hitting the spacebar allows the player to jump (currently an infinite amount of times). One of the next things that I plan to implement is limiting the player's jumps, so that they can perform a double jump, but nothing more.
public void Update() { // Jump if (Input.GetKeyDown(moveJump)) { rigidbody.velocity = new Vector2 (rigidbody.velocity.x, jumpVelocity); } }
The code which makes the player jump, currently lacking a check to see if the player is on the ground to stop them jumping an infinite amount of times.
The player character has a BoxCollider2D which allows them to stand in the environment, and this collider has a physics material with a fairly strong friction value. This makes the player come to a stop fairly quickly when they stop pressing a movement key, while also not making the movement feel super jarring like it would if they stopped dead still after releasing a key. However, with only one collider attached to the player, an issue arose where if you held a movement key against a wall, you would be able to stay pressed against the wall and not fall. While this would be a suitable behaviour in some games, I don't think that it is suitable for what I have in mind for this game, and so this problem required solving.
Trying to move right when pressed against the wall allowed the player to cling there indefinitely.
This problem troubled me, and I didn't really know how to fix it for a while. I knew that I had to change the friction on the player character to 0 to stop this behaviour, but doing this also meant that the player would slide along the ground as if it were ice. Eventually, I solved this problem by adding another box collider to the player character, which is slightly wider than the original collider, and attached a new physics object with a friction value of 0 to it. This means that the new frictionless collider will be the only collider to collide with walls, while the other box collider is the only collider to touch the ground. This makes the player slide down walls due to gravity, while also not making the floor an ice skating rink.
The player no longer clings to walls while trying to move towards them.
While this all worked fine in the Unity editor, after uploading the build here, the frictionless collider was taking precedence over the other collider in all situations, making the player slide everywhere. This was resolved with a slight change to the height of the frictionless BoxCollider2D, making sure that the other collider is the one that hits the ground.
Example Scene
The scene that is on display in this build of the game demonstrates the use of tilemaps to create an environment that the player can manoeuvre around. While it doesn't look spectacular at the moment, it allows the player to get used to the simple controls that the game currently has.
Among the things present in the scene are tall walls that the player must double jump up, pits that they must jump over, and also ledges or platforms that they must jump up to reach the "end" of the level.
The player jumping over a bottomless pit, before falling into the next one.
By making use of a CinemachineConfiner, I have set boundaries that the camera will not go outside of. This allows me to "guide" the camera as the player moves through the level, such as at the bottomless pits. By using boundaries, I can stop the camera from going to far down and revealing to the player that the bottomless pits aren't actually bottomless.
The yellow line is the boundary for the camera, so it will not display anything under that line.
However, one difficulty I encountered while using the CinemachineConfiner, was sometimes the camera would jarringly jump from one position to another occasionally. I'm not really sure how to explain this in text and I didn't capture any footage of this before fixing it, but the camera feels much smoother now. In the future, when the player falls down a hole like these, the camera will fade to black and they will be sent back to a respawn point somewhere close by.
Full image of the current level, as well as the camera boundary line.
Next Steps
- Limiting the player to only a double jump
- Add the basic 'shoot' attack
- Add player health and other variables
- Add enemies which attack the player
Get Ability Game
Ability Game
Defeat enemies and traverse your environment by making use of a set of powerful abilities.
Status | In development |
Author | ow514073 |
More posts
- Final Build - User GuideMay 30, 2021
- Final Build - Documentation DevlogMay 30, 2021
- Final Build - UI, Updated Aesthetics, Updated Game Modes and more....May 30, 2021
- Build 5 - Testing, Updated Aesthetics, Enemy Interaction and MovementMay 23, 2021
- Build 4 - Updated Player Abilities and Basic SpritesMay 20, 2021
- Build 3 - Basic Player AbilitiesMay 10, 2021
- Build 2 - Enemies, Player Animation and Updated Player MovementMay 02, 2021
- Assignment 2 DevlogApr 16, 2021
Leave a comment
Log in with itch.io to leave a comment.